[ST] OOP các kiến thức chung lập trình hướng đối tượng
Posted: Thu Jul 07, 2016 7:46 am
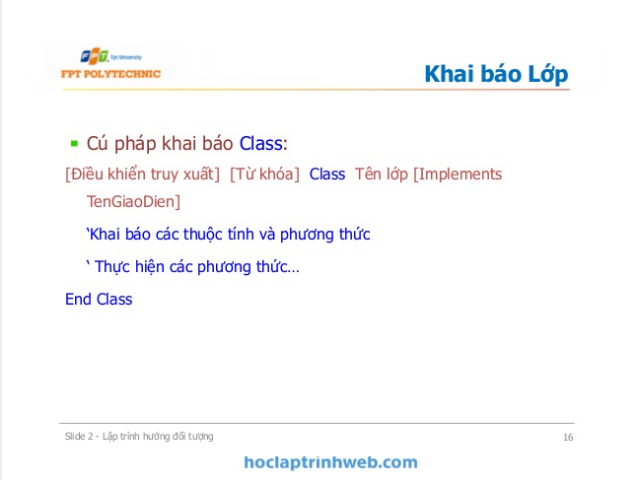
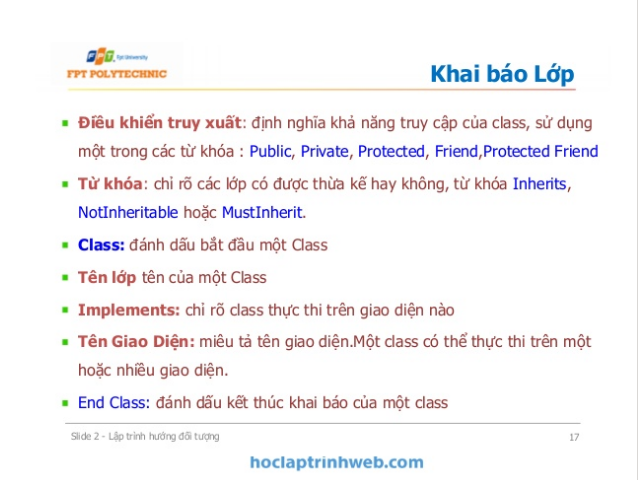
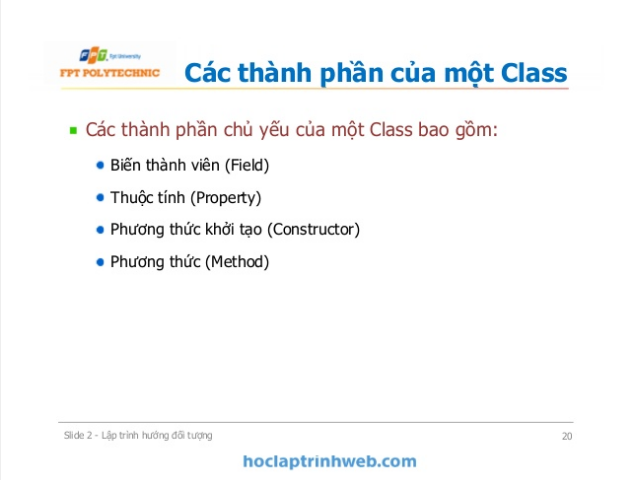
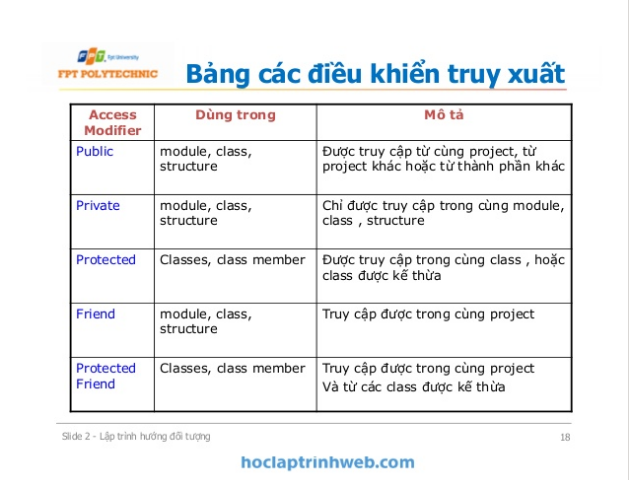
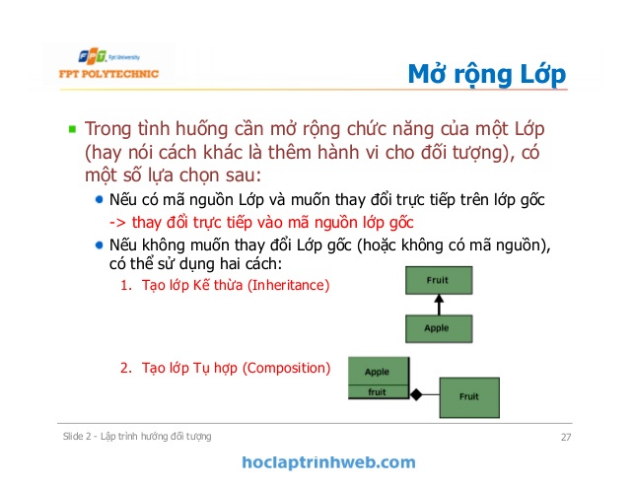
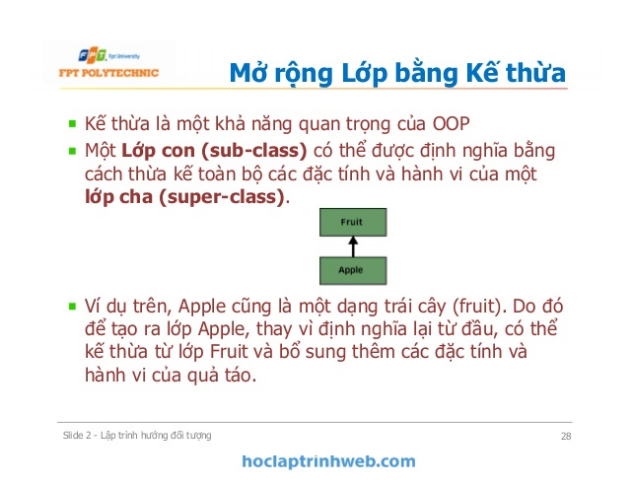
A short text to describe your forum
https://tthlan.info/forum/
Code: Select all
Example:
<?php
class GrandPa
{
public $name='Mark Henry'; // A public variable
}
class Daddy extends GrandPa // Inherited class
{
function displayGrandPaName()
{
return $this->name; // The public variable will be available to the inherited class
}
}
// Inherited class Daddy wants to know Grandpas Name
$daddy = new Daddy;
echo $daddy->displayGrandPaName(); // Prints 'Mark Henry'
// Public variables can also be accessed outside of the class!
$outsiderWantstoKnowGrandpasName = new GrandPa;
echo $outsiderWantstoKnowGrandpasName->name; // Prints 'Mark Henry'
Code: Select all
Example:
<?php
class GrandPa
{
protected $name = 'Mark Henry';
}
class Daddy extends GrandPa
{
function displayGrandPaName()
{
return $this->name;
}
}
$daddy = new Daddy;
echo $daddy->displayGrandPaName(); // Prints 'Mark Henry'
$outsiderWantstoKnowGrandpasName = new GrandPa;
echo $outsiderWantstoKnowGrandpasName->name; // Results in a Fatal Error
The exact error will be this:
PHP Fatal error: Cannot access protected property GrandPa::$name
Code: Select all
Example:
<?php
class GrandPa
{
private $name = 'Mark Henry';
}
class Daddy extends GrandPa
{
function displayGrandPaName()
{
return $this->name;
}
}
$daddy = new Daddy;
echo $daddy->displayGrandPaName(); // Results in a Notice
$outsiderWantstoKnowGrandpasName = new GrandPa;
echo $outsiderWantstoKnowGrandpasName->name; // Results in a Fatal Error
The exact error messages will be:
Notice: Undefined property: Daddy::$name
Fatal error: Cannot access private property GrandPa::$name
Code: Select all
//** Ví dụ 1
//** Abstract class
public abstract class Employee
{
//Khai báo Properties
public abstract String ID
{
get;
set;
}
//Khai báo Method
public abstract String Add();
public abstract String Update();
}
}
//** Interface
public interface IEmployee
{
//Khai báo Properties
String ID
{
get;
set;
}
//Khai báo Method
String Add();
String Update();
}
}
Code: Select all
//** Ví dụ 2
//** Abstract class
public abstract class Employee
{
//Khai báo biến hằng (field and constant)
public String id;
public String name;
//Khai báo Properties
public abstract String ID
{
get;
set;
}
//Khai báo Method
protected abstract String Add();
//Method đã triển khai không dùng abstract
public String Update()
{
return "Update";
}
}
}
//** Interface
public interface IEmployee
{
//Khai báo Properties
String ID
{
get;
set;
}
//Khai báo Method
String Add();
String Update();
}
}
Code: Select all
//** Sử dụng Abstract class và Interface ở ví dụ 2
//** Lớp dẫn xuất Abstract class
public class Employee1 : Employee
{
//Không cần khai báo lại biến hằng.
//Triển khai Properties với từ khóa override
public override String ID
{
get{ return id; }
set{ id = value; }
}
//Triển khai Method với access modifier như ở Abstract class.
protected override String Add()
{
return "Add";
}
//Không cần triển khai lại Update() mà chỉ việc sử dụng.
// Phần triển khai của Employee1
...
}
//** Lớp dẫn xuất Interface
public class Employee1 : IEmployee
{
// Biến hằng được định nghĩa ở đây.
protected String id;
protected String name;
// Triển khai Properties
String ID
{
get{ return id; }
set{ id = value; }
}
//Triển khai tất cả các Method có ở Interface
public String Add()
{
return "Add";
}
public String Update()
{
return "Update";
}
// Phần triển khai của Employee1
...
}